Interpreter
Objective
Given a programming language, it defines a representation with an interpreter that is used for the statements.
Function
Define a grammar for a given language, as well as the tools needed to interpret it.
Structure
As shown in figure 1
- Client: Actor who triggers the performance of the interpreter.
- Context: Object with global information that will be used by the interpreter to read and store global information among all the classes that make up the pattern, this is sent to the interpreter who replicates it throughout the structure.
- AbstractExpression: Interface that defines the minimum structure of an expression.
- TerminalExpression: Refers to expressions that have no more continuity and when evaluated or interpreted they end the execution of that branch. These expressions mark the end of the execution of a sub-tree of the expression.
- ExpressionsNoTerminal: These are composite expressions and within them there are more expressions to be evaluated. These structures are interpreted using recursion until arriving at a Terminal expression.
The structure that meets this pattern is shown in Figure 1
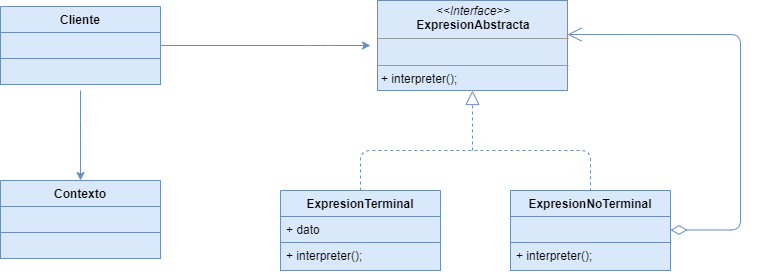
Figure 1: UML Diagram Interpreter Pattern
Applications
The use of the Interpreter pattern is recommended when:
- You want to interpret a grammar, and this is not very complex.
- The expected efficiency of the application is not demanding.
Design Patterns Collaborators
- Tree-structured statements are generally implemented as a composite.
- The flyweight pattern shows how to share terminal symbols within the abstract tree syntax.
- An Iterator pattern can be used to navigate the structure.
- The visitor pattern can be used to maintain the behavior of each node in the tree structure.
Scope of action
Applied at the object level.
Problem
To execute various commands or instructions, these are defined in a basic structure so that the application interprets them correctly; by means of an object that interprets the sentences by means of if or Switch, case: conditions, which considerably complicates programming.
Solution
The Interpreter design pattern defines the instructions as objects, which allows each implemented instruction to identify itself as a statement or another one through a tree structure analysis; all this process through an interface.
Diagram or Implementation
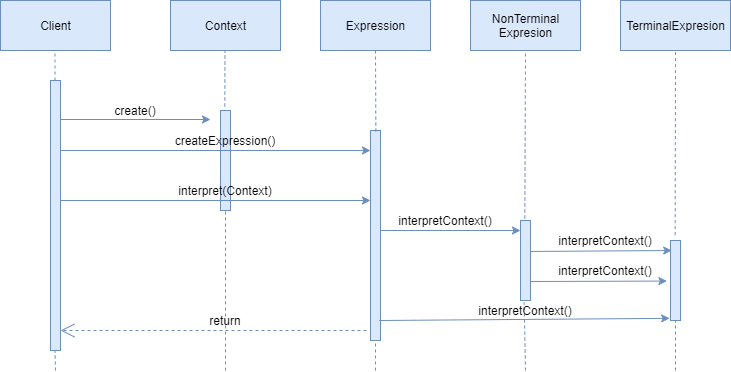
Figure 2: UML Diagram Interpreter Pattern
Figure 2 explains the behaviour of the pattern by means of a sequence diagram.
- The client class creates the context for the execution of the interpreter component.
- The client class creates or obtains the expression to be evaluated.
- The client class requests the interpretation of the expression from the interpreter component and sends it the context.
- The Expression calls the Non-Terminal Expressions it contains.
- The Non-Terminal Expression calls all Terminal Expressions.
- The Root Expression requests the interpretation of a Terminal Expression.
- The expression is fully evaluated and you have a result from the interpretation of all terminal and non-terminal expressions.
Implementations of the Interpreter pattern:
from __future__ import annotations
from abc import ABC, abstractmethod, abstractproperty
from typing import Any
class Builder(ABC):
"""
The Builder interface specifies methods for creating the different parts of
the Product objects.
"""
@abstractproperty
def product(self) -> None:
pass
@abstractmethod
def produce_part_a(self) -> None:
pass
@abstractmethod
def produce_part_b(self) -> None:
pass
@abstractmethod
def produce_part_c(self) -> None:
pass
class ConcreteBuilder1(Builder):
"""
The Concrete Builder classes follow the Builder interface and provide
specific implementations of the building steps. Your program may have
several variations of Builders, implemented differently.
"""
def __init__(self) -> None:
"""
A fresh builder instance should contain a blank product object, which is
used in further assembly.
"""
self.reset()
def reset(self) -> None:
self._product = Product1()
@property
def product(self) -> Product1:
"""
Concrete Builders are supposed to provide their own methods for
retrieving results. That's because various types of builders may create
entirely different products that don't follow the same interface.
Therefore, such methods cannot be declared in the base Builder interface
(at least in a statically typed programming language).
Usually, after returning the end result to the client, a builder
instance is expected to be ready to start producing another product.
That's why it's a usual practice to call the reset method at the end of
the `getProduct` method body. However, this behavior is not mandatory,
and you can make your builders wait for an explicit reset call from the
client code before disposing of the previous result.
"""
product = self._product
self.reset()
return product
def produce_part_a(self) -> None:
self._product.add("PartA1")
def produce_part_b(self) -> None:
self._product.add("PartB1")
def produce_part_c(self) -> None:
self._product.add("PartC1")
class Product1():
"""
It makes sense to use the Builder pattern only when your products are quite
complex and require extensive configuration.
Unlike in other creational patterns, different concrete builders can produce
unrelated products. In other words, results of various builders may not
always follow the same interface.
"""
def __init__(self) -> None:
self.parts = []
def add(self, part: Any) -> None:
self.parts.append(part)
def list_parts(self) -> None:
print(f"Product parts: {', '.join(self.parts)}", end="")
class Director:
"""
The Director is only responsible for executing the building steps in a
particular sequence. It is helpful when producing products according to a
specific order or configuration. Strictly speaking, the Director class is
optional, since the client can control builders directly.
"""
def __init__(self) -> None:
self._builder = None
@property
def builder(self) -> Builder:
return self._builder
@builder.setter
def builder(self, builder: Builder) -> None:
"""
The Director works with any builder instance that the client code passes
to it. This way, the client code may alter the final type of the newly
assembled product.
"""
self._builder = builder
"""
The Director can construct several product variations using the same
building steps.
"""
def build_minimal_viable_product(self) -> None:
self.builder.produce_part_a()
def build_full_featured_product(self) -> None:
self.builder.produce_part_a()
self.builder.produce_part_b()
self.builder.produce_part_c()
if __name__ == "__main__":
"""
The client code creates a builder object, passes it to the director and then
initiates the construction process. The end result is retrieved from the
builder object.
"""
director = Director()
builder = ConcreteBuilder1()
director.builder = builder
print("Standard basic product: ")
director.build_minimal_viable_product()
builder.product.list_parts()
print("\n")
print("Standard full featured product: ")
director.build_full_featured_product()
builder.product.list_parts()
print("\n")
# Remember, the Builder pattern can be used without a Director class.
print("Custom product: ")
builder.produce_part_a()
builder.produce_part_b()
builder.product.list_parts()
namespace RefactoringGuru\Builder\Conceptual;
/**
* The Builder interface specifies methods for creating the different parts of
* the Product objects.
*/
interface Builder
{
public function producePartA(): void;
public function producePartB(): void;
public function producePartC(): void;
}
/**
* The Concrete Builder classes follow the Builder interface and provide
* specific implementations of the building steps. Your program may have several
* variations of Builders, implemented differently.
*/
class ConcreteBuilder1 implements Builder
{
private $product;
/**
* A fresh builder instance should contain a blank product object, which is
* used in further assembly.
*/
public function __construct()
{
$this->reset();
}
public function reset(): void
{
$this->product = new Product1();
}
/**
* All production steps work with the same product instance.
*/
public function producePartA(): void
{
$this->product->parts[] = "PartA1";
}
public function producePartB(): void
{
$this->product->parts[] = "PartB1";
}
public function producePartC(): void
{
$this->product->parts[] = "PartC1";
}
/**
* Concrete Builders are supposed to provide their own methods for
* retrieving results. That's because various types of builders may create
* entirely different products that don't follow the same interface.
* Therefore, such methods cannot be declared in the base Builder interface
* (at least in a statically typed programming language). Note that PHP is a
* dynamically typed language and this method CAN be in the base interface.
* However, we won't declare it there for the sake of clarity.
*
* Usually, after returning the end result to the client, a builder instance
* is expected to be ready to start producing another product. That's why
* it's a usual practice to call the reset method at the end of the
* `getProduct` method body. However, this behavior is not mandatory, and
* you can make your builders wait for an explicit reset call from the
* client code before disposing of the previous result.
*/
public function getProduct(): Product1
{
$result = $this->product;
$this->reset();
return $result;
}
}
/**
* It makes sense to use the Builder pattern only when your products are quite
* complex and require extensive configuration.
*
* Unlike in other creational patterns, different concrete builders can produce
* unrelated products. In other words, results of various builders may not
* always follow the same interface.
*/
class Product1
{
public $parts = [];
public function listParts(): void
{
echo "Product parts: " . implode(', ', $this->parts) . "\n\n";
}
}
/**
* The Director is only responsible for executing the building steps in a
* particular sequence. It is helpful when producing products according to a
* specific order or configuration. Strictly speaking, the Director class is
* optional, since the client can control builders directly.
*/
class Director
{
/**
* @var Builder
*/
private $builder;
/**
* The Director works with any builder instance that the client code passes
* to it. This way, the client code may alter the final type of the newly
* assembled product.
*/
public function setBuilder(Builder $builder): void
{
$this->builder = $builder;
}
/**
* The Director can construct several product variations using the same
* building steps.
*/
public function buildMinimalViableProduct(): void
{
$this->builder->producePartA();
}
public function buildFullFeaturedProduct(): void
{
$this->builder->producePartA();
$this->builder->producePartB();
$this->builder->producePartC();
}
}
/**
* The client code creates a builder object, passes it to the director and then
* initiates the construction process. The end result is retrieved from the
* builder object.
*/
function clientCode(Director $director)
{
$builder = new ConcreteBuilder1();
$director->setBuilder($builder);
echo "Standard basic product:\n";
$director->buildMinimalViableProduct();
$builder->getProduct()->listParts();
echo "Standard full featured product:\n";
$director->buildFullFeaturedProduct();
$builder->getProduct()->listParts();
// Remember, the Builder pattern can be used without a Director class.
echo "Custom product:\n";
$builder->producePartA();
$builder->producePartC();
$builder->getProduct()->listParts();
}
$director = new Director();
clientCode($director);
/* "Product" */
class Pizza {
private String dough = "";
private String sauce = "";
private String topping = "";
public void setDough(String dough) {
this.dough = dough;
}
public void setSauce(String sauce) {
this.sauce = sauce;
}
public void setTopping(String topping) {
this.topping = topping;
}
}
/* "Abstract Builder" */
abstract class PizzaBuilder {
protected Pizza pizza;
public Pizza getPizza() {
return pizza;
}
public void createNewPizzaProduct() {
pizza = new Pizza();
}
public abstract void buildDough();
public abstract void buildSauce();
public abstract void buildTopping();
}
/* "ConcreteBuilder" */
class HawaiianPizzaBuilder extends PizzaBuilder {
public void buildDough() {
pizza.setDough("cross");
}
public void buildSauce() {
pizza.setSauce("mild");
}
public void buildTopping() {
pizza.setTopping("ham+pineapple");
}
}
/* "ConcreteBuilder" */
class SpicyPizzaBuilder extends PizzaBuilder {
public void buildDough() {
pizza.setDough("pan baked");
}
public void buildSauce() {
pizza.setSauce("hot");
}
public void buildTopping() {
pizza.setTopping("pepperoni+salami");
}
}
/* "Director" */
class Waiter {
private PizzaBuilder pizzaBuilder;
public void setPizzaBuilder(PizzaBuilder pb) {
pizzaBuilder = pb;
}
public Pizza getPizza() {
return pizzaBuilder.getPizza();
}
public void constructPizza() {
pizzaBuilder.createNewPizzaProduct();
pizzaBuilder.buildDough();
pizzaBuilder.buildSauce();
pizzaBuilder.buildTopping();
}
}
/* A customer ordering a pizza. */
public class PizzaBuilderDemo {
public static void main(String[] args) {
Waiter waiter = new Waiter();
PizzaBuilder hawaiianPizzabuilder = new HawaiianPizzaBuilder();
PizzaBuilder spicyPizzaBuilder = new SpicyPizzaBuilder();
waiter.setPizzaBuilder( hawaiianPizzabuilder );
waiter.constructPizza();
Pizza pizza = waiter.getPizza();
}
}