Composite
Objective
Forming hierarchical structures so that individual components can be treated as well as groups of components The operations Typical components include add, delete, display, find and group.
Function
Handle composite objects as if they were simple.
Structure
The Component interface describes operations that are common to simple and complex elements. The Client works with all the elements to through the component's interface. As a result, the customer can work in the same way with simple or complex elements.
The structure that meets this pattern is shown in Figure 1
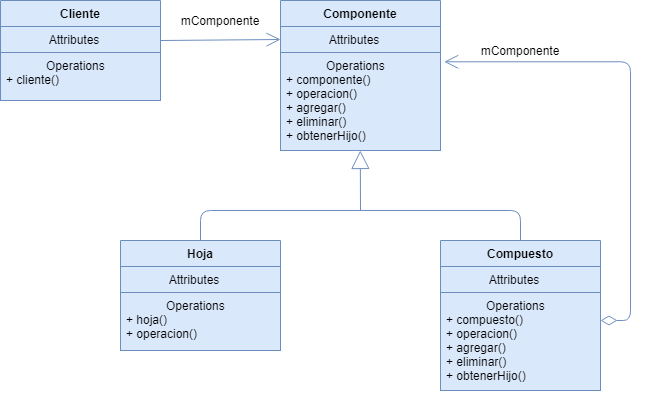
Figure 1: UML Diagram Composite Pattern
Applications
The use of the Composite pattern is recommended in the following cases:
- You want the client classes to handle all objects in a hierarchical structure, not knowing whether they are managing a single object or a composite one.
- You want to represent entire object hierarchies or just a part of them of them.
Design Patterns Collaborators
- A composite is generally ideal for Chain of Responsibility responsibilities.
- A decorator pattern usually uses a composite.
- The iterator pattern can be used to go through the composites.
Scope of action
Applied at the object level.
Problem
To create objects, individuals or a grouping, similar to a tree structure with compound or single nodes; lists should be used The difficulty in these cases, however, is knowing how to recognize a composite object or a simple one, in order to determine which operations to apply in each case.
Solution
The composite design pattern allows individual objects to be referenced or sheet, using the same "Component" interface that directly executes the operation in case of a sheet object, while in case of a composite object it replicates all operations to each of the component or sheet objects.
Diagram or Implementation
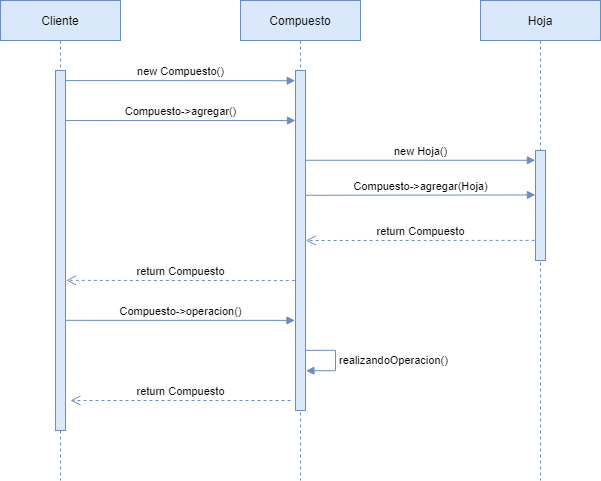
Figure 2: UML Diagram Composite Pattern
Figure 2 explains the behaviour of the Composite pattern by means of a sequence diagram.
- The client class perform an action on the CompositeA class.
- CompositeA class in turn performs an action on CompositeB class.
- Class CompositeB performs an action on class LeafA and class LeafB and the result is returned to class CompositeA.
- The CompositeA class spreads the action on LeafC, which returns a result.
- The CompositeA class obtains a final result after the evaluation of all the structure and the client class gets a result.
Study Cases
Drawing Editor System
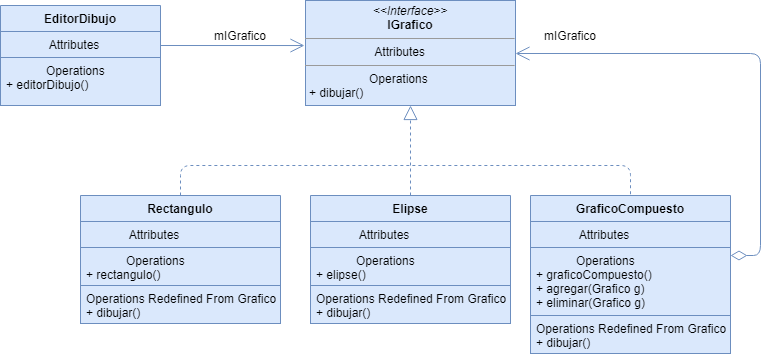
Figure 3: UML Diagram Drawing Editor System
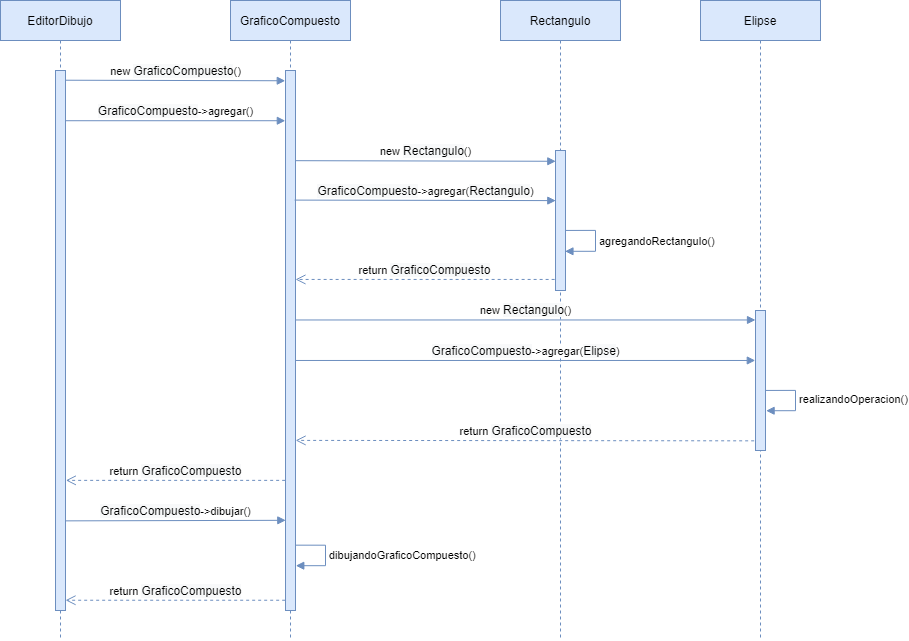
Figure 4: UML Diagram Drawing Editor System
Tourist Reservation System
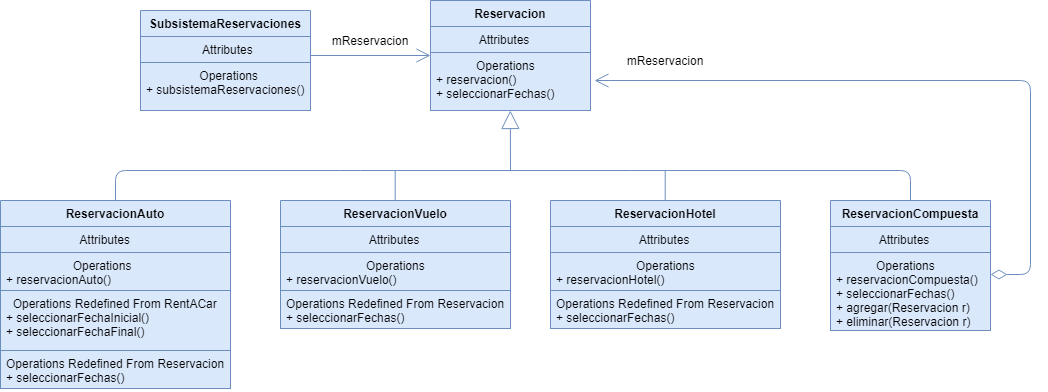
Figure 5: UML Diagram Tourist Reservation System
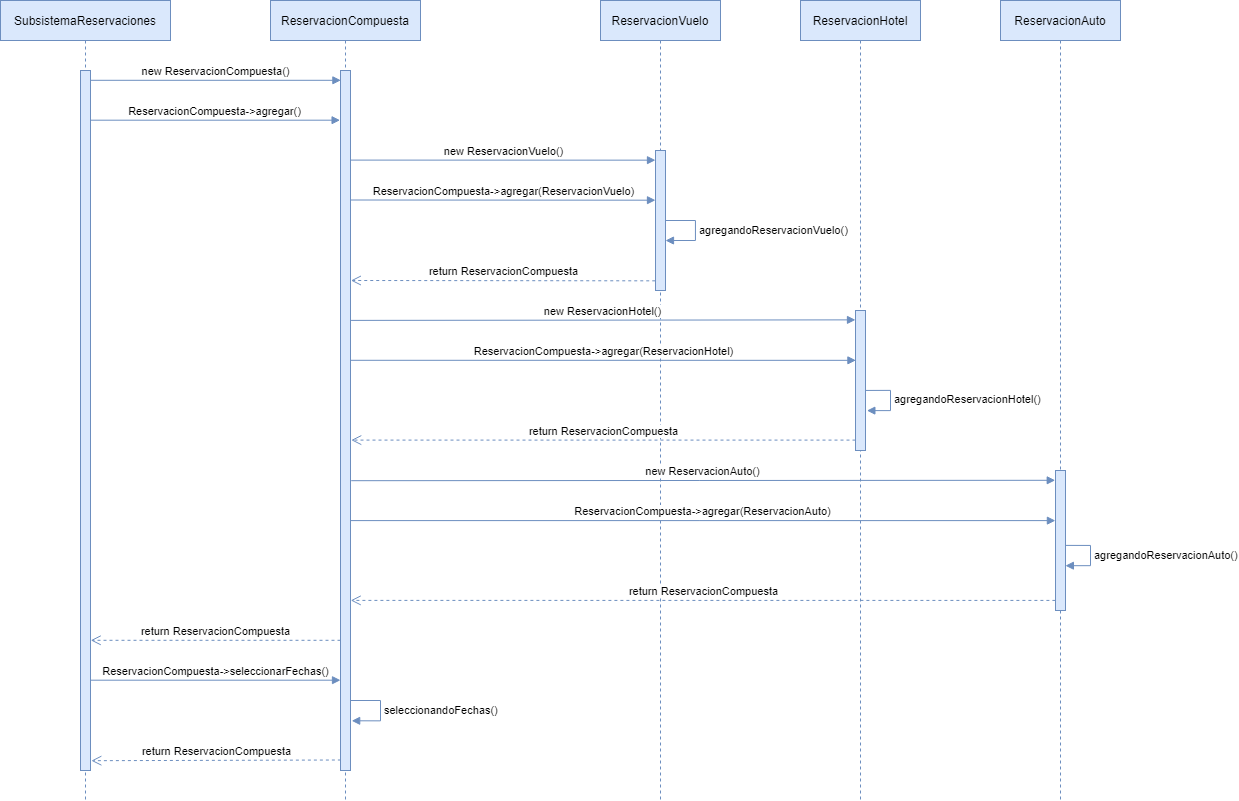
Figure 6: UML Diagram Tourist Reservation System
Implementations of the composite pattern:
"""
Compose objects into tree structures to represent part-whole
hierarchies. Composite lets clients treat individual objects and
compositions of objects uniformly.
"""
import abc
class Component(metaclass=abc.ABCMeta):
"""
Declare the interface for objects in the composition.
Implement default behavior for the interface common to all classes,
as appropriate.
Declare an interface for accessing and managing its child
components.
Define an interface for accessing a component's parent in the
recursive structure, and implement it if that's appropriate
(optional).
"""
@abc.abstractmethod
def operation(self):
pass
class Composite(Component):
"""
Define behavior for components having children.
Store child components.
Implement child-related operations in the Component interface.
"""
def __init__(self):
self._children = set()
def operation(self):
for child in self._children:
child.operation()
def add(self, component):
self._children.add(component)
def remove(self, component):
self._children.discard(component)
class Leaf(Component):
"""
Represent leaf objects in the composition. A leaf has no children.
Define behavior for primitive objects in the composition.
"""
def operation(self):
pass
def main():
leaf = Leaf()
composite = Composite()
composite.add(leaf)
composite.operation()
if __name__ == "__main__":
main()
abstract class OnTheBookShelf {
abstract function getBookInfo($previousBook);
abstract function getBookCount();
abstract function setBookCount($new_count);
abstract function addBook($oneBook);
abstract function removeBook($oneBook);
}
class OneBook extends OnTheBookShelf {
private $title;
private $author;
function __construct($title, $author) {
$this->title = $title;
$this->author = $author;
}
function getBookInfo($bookToGet) {
if (1 == $bookToGet) {
return $this->title." by ".$this->author;
} else {
return FALSE;
}
}
function getBookCount() {
return 1;
}
function setBookCount($newCount) {
return FALSE;
}
function addBook($oneBook) {
return FALSE;
}
function removeBook($oneBook) {
return FALSE;
}
}
class SeveralBooks extends OnTheBookShelf {
private $oneBooks = array();
private $bookCount;
public function __construct() {
$this->setBookCount(0);
}
public function getBookCount() {
return $this->bookCount;
}
public function setBookCount($newCount) {
$this->bookCount = $newCount;
}
public function getBookInfo($bookToGet) {
if ($bookToGet <= $this->bookCount) {
return $this->oneBooks[$bookToGet]->getBookInfo(1);
} else {
return FALSE;
}
}
public function addBook($oneBook) {
$this->setBookCount($this->getBookCount() + 1);
$this->oneBooks[$this->getBookCount()] = $oneBook;
return $this->getBookCount();
}
public function removeBook($oneBook) {
$counter = 0;
while (++$counter <= $this->getBookCount()) {
if ($oneBook->getBookInfo(1) ==
$this->oneBooks[$counter]->getBookInfo(1)) {
for ($x = $counter; $x < $this->getBookCount(); $x++) {
$this->oneBooks[$x] = $this->oneBooks[$x + 1];
}
$this->setBookCount($this->getBookCount() - 1);
}
}
return $this->getBookCount();
}
}
writeln("BEGIN TESTING COMPOSITE PATTERN");
writeln('');
$firstBook = new OneBook('Core PHP Programming, Third Edition', 'Atkinson and Suraski');
writeln('(after creating first book) oneBook info: ');
writeln($firstBook->getBookInfo(1));
writeln('');
$secondBook = new OneBook('PHP Bible', 'Converse and Park');
writeln('(after creating second book) oneBook info: ');
writeln($secondBook->getBookInfo(1));
writeln('');
$thirdBook = new OneBook('Design Patterns', 'Gamma, Helm, Johnson, and Vlissides');
writeln('(after creating third book) oneBook info: ');
writeln($thirdBook->getBookInfo(1));
writeln('');
$books = new SeveralBooks();
$booksCount = $books->addBook($firstBook);
writeln('(after adding firstBook to books) SeveralBooks info : ');
writeln($books->getBookInfo($booksCount));
writeln('');
$booksCount = $books->addBook($secondBook);
writeln('(after adding secondBook to books) SeveralBooks info : ');
writeln($books->getBookInfo($booksCount));
writeln('');
$booksCount = $books->addBook($thirdBook);
writeln('(after adding thirdBook to books) SeveralBooks info : ');
writeln($books->getBookInfo($booksCount));
writeln('');
$booksCount = $books->removeBook($firstBook);
writeln('(after removing firstBook from books) SeveralBooks count : ');
writeln($books->getBookCount());
writeln('');
writeln('(after removing firstBook from books) SeveralBooks info 1 : ');
writeln($books->getBookInfo(1));
writeln('');
writeln('(after removing firstBook from books) SeveralBooks info 2 : ');
writeln($books->getBookInfo(2));
writeln('');
writeln('END TESTING COMPOSITE PATTERN');
function writeln($line_in) {
echo $line_in."
";
}
// 1. "lowest common denominator"
interface Component {
void traverse();
}
// 2. "Isa" relationship
class Primitive implements Component {
private int value;
public Primitive(int val) {
value = val;
}
public void traverse() {
System.out.print( value + " " );
}
}
// 2. "Isa" relationship
abstract class Composite implements Component {
// 3. Couple to interface
private Component[] children = new Component[9];
private int total = 0;
private int value;
public Composite(int val) {
value = val;
}
// 3. Couple to interface
public void add(Component c) {
children[total++] = c;
}
public void traverse() {
System.out.print(value + " ");
for (int i=0; i < total; i++) {
// 4. Delegation and polymorphism
children[i].traverse();
}
}
}
// Two different kinds of "container" classes. Most of the
// "meat" is in the Composite base class.
class Row extends Composite {
public Row(int val) {
super(val);
}
public void traverse() {
System.out.print("Row");
super.traverse();
}
}
class Column extends Composite {
public Column(int val) {
super(val);
}
public void traverse() {
System.out.print("Col");
super.traverse();
}
}
public class CompositeDemo {
public static void main( String[] args ) {
Composite first = new Row( 1 );
Composite second = new Column( 2 );
Composite third = new Column( 3 );
Composite fourth = new Row( 4 );
Composite fifth = new Row( 5 );
first.add(second);
first.add(third);
third.add(fourth);
third.add(fifth);
first.add(new Primitive(6));
second.add(new Primitive(7));
third.add(new Primitive(8));
fourth.add(new Primitive(9));
fifth.add(new Primitive(10));
first.traverse();
}
}